jQuery 元素尺寸
在本教程中,您将学习如何使用 jQuery 获取或设置元素框的尺寸,例如宽度和高度。
了解 jQuery 尺寸
jQuery 提供了几种方法,例如 height()
, innerHeight()
, outerHeight()
, width()
, innerWidth()
和 outerWidth()
来获取和设置元素的 CSS 尺寸。 查看下图以了解这些方法如何计算元素框的尺寸。
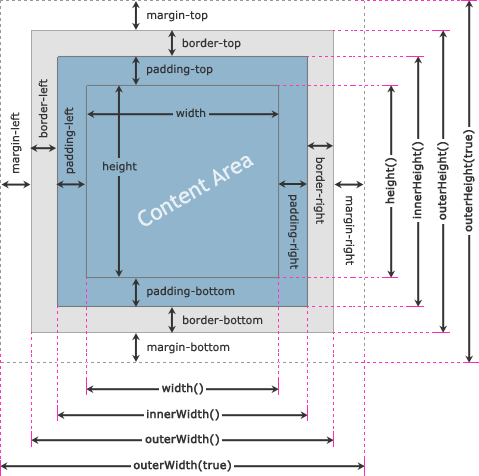
jQuery width()
和 height()
方法
jQuery width()
和 height()
方法分别获取或设置元素的 宽度
和 高度
。 此宽度和高度不包括元素上的 填充
, 边框
和 margin
。 以下示例将返回 <div>
元素的宽度和高度。
<script>
$(document).ready(function(){
$("button").click(function(){
var divWidth = $("#box").width();
var divHeight = $("#box").height();
$("#result").html("Width: " + divWidth + ", " + "Height: " + divHeight);
});
});
</script>
同样,您可以通过将值作为参数包含在 width()
和 height()
方法中来设置元素的宽度和高度。 该值可以是字符串(数字和单位,例如 100px、20em 等)或数字。 下面的示例将 <div>
元素的宽度分别设置为 400 像素和高度为 300 像素。
<script>
$(document).ready(function(){
$("button").click(function(){
$("#box").width(400).height(300);
});
});
</script>
jQuery innerWidth()
和 innerHeight()
方法
jQuery innerWidth()
和 innerHeight()
方法分别获取或设置元素的 内部宽度 和 内部高度。 这个内部宽度和高度包括 填充(padding)
但不包括元素上的 边框(border)
和 边距(margin)
。 以下示例将在单击按钮时返回 <div>
元素的内部宽度和高度。
<script>
$(document).ready(function(){
$("button").click(function(){
var divWidth = $("#box").innerWidth();
var divHeight = $("#box").innerHeight();
$("#result").html("Inner Width: " + divWidth + ", " + "Inner Height: " + divHeight);
});
});
</script>
同样,您可以通过将值作为参数传递给 innerWidth()
和 innerHeight()
方法来设置元素的内部宽度和高度。 这些方法仅更改元素内容区域的宽度或高度以匹配指定值。
例如,如果元素的当前宽度为 300 像素,并且左右填充之和等于 50 像素,则将内部宽度设置为 400 像素后元素的新宽度为 350 像素,即 新宽度 = 内部宽度 - 水平填充
。 同样,您可以在设置内部高度的同时估计高度的变化。
<script>
$(document).ready(function(){
$("button").click(function(){
$("#box").innerWidth(400).innerHeight(300);
});
});
</script>
jQuery outerWidth()
和 outerHeight()
方法
jQuery outerWidth()
和 outerHeight()
方法分别获取或设置元素的外部宽度和外部高度。 这个外部宽度和高度包括 填充(padding)
和 边框(border)
但不包括元素上的 边距(margin)
。 以下示例将在单击按钮时返回 <div>
元素的外部宽度和高度。
<script>
$(document).ready(function(){
$("button").click(function(){
var divWidth = $("#box").outerWidth();
var divHeight = $("#box").outerHeight();
$("#result").html("Outer Width: " + divWidth + ", " + "Outer Height: " + divHeight);
});
});
</script>
您还可以获得包含 padding
和 border
以及元素的 margin
的外部宽度和高度。 为此,只需为外部宽度方法指定 true
参数,例如 outerWidth(true)
和 outerHeight(true)
。
<script>
$(document).ready(function(){
$("button").click(function(){
var divWidth = $("#box").outerWidth(true);
var divHeight = $("#box").outerHeight(true);
$("#result").html("Outer Width: " + divWidth + ", " + "Outer Height: " + divHeight);
});
});
</script>
同样,您可以通过将值作为参数传递给 outerWidth()
和 outerHeight()
方法来设置元素的外部宽度和高度。 这些方法仅更改元素内容区域的宽度或高度以匹配指定值,如 innerWidth()
和 innerHeight()
方法。
例如,如果元素的当前宽度为 300 像素,左右填充之和等于 50 像素,则左右边框的宽度之和比新的宽度为 20 像素 将外部宽度设置为 400 像素后的元素为 330 像素,即 新宽度 = 外部宽度 - (水平填充 + 水平边框)
。 同样,您可以在设置外部高度时估计高度的变化。
<script>
$(document).ready(function(){
$("button").click(function(){
$("#box").outerWidth(400).outerHeight(300);
});
});
</script>